Sveltekit basic fetching?
Heyy, ich habe tutorials für sveltekit angeschaut und es wurde ein leichtes beispiel für einen fetch gezeigt, der aber schon zwei jahre alt ist und nicht funktioniert chatgpt konnte mir auch nicht weiterhelfen. Vielen Dank im vorraus.
<script context="module">
export async function load({ fetch }) {
const res = await fetch('https://jsonplaceholder.typicode.com/posts')
const guides = await res.json()
if (res.ok) {
return {
props: {
guides
}
}
}
return {
status: res.status,
error: new Error('Could not fetch the guides')
}
}
</script>
<script>
export let guides
</script>
<div class="guides">
<ul>
{#each guides as guide}
<li>
<a href='/'>{guide.title}</a>
</li>
{/each}
</ul>
</div>
<style>
.guides {
margin-top: 20px;
}
ul {
list-style-type: none;
padding: 0;
}
a {
display: inline-block;
margin-top: 10px;
padding: 10px;
border: 1px dotted rgba(255,255,255,0.2);
}
</style>
die Fehlermeldung lautet: TypeError: undefined is not iterable (cannot read property Symbol(Symbol.iterator))
1 Antwort
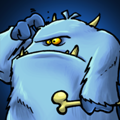
Von gutefrage auf Grund seines Wissens auf einem Fachgebiet ausgezeichneter Nutzer
Webseite, Webentwicklung, Programmieren & Softwareentwicklung
Das Problem liegt nicht im fetch-Request, sondern darin, wie du versuchst, die Daten weiterzugeben.
Ein sehr einfaches Beispiel kann zunächst so aussehen:
<script>
import { onMount } from "svelte";
let guides = [];
onMount(async () => {
const response = await fetch("https://jsonplaceholder.typicode.com/posts");
if (response.ok) {
guides = await response.json();
}
});
</script>
<div class="guides">
<ul>
{#each guides as guide}
<li>{guide.title}</li>
{/each}
</ul>
</div>
Für die Verwendung von load-Funktionen lies zuerst in der Dokumentation, wie es funktioniert.
Beispielimplementation:
page.js
export async function load({ fetch }) {
const response = await fetch("https://jsonplaceholder.typicode.com/posts");
if (response.ok) {
const result = await response.json();
return { guides };
}
/* otherwise do some error handling ... */
}
page.svelte
<script>
export let data;
</script>
<div class="guides">
<ul>
{#each data.guides as guide}
<li>{guide.title}</li>
{/each}
</ul>
</div>