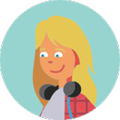
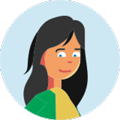
Antwort
So könntest du beispielsweise deine PHP Funktionen mit HTML verknüpfen und dir deine Einträge in Tabellen anzeigen
index.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.4.1/dist/css/bootstrap.min.css">
<title>Document</title>
</head>
<body>
<h1>CusSysMgmt</h1>
<div class="contain-to-grid">
<nav class="top-bar" data-topbar>
<section class="top-bar-section">
<ul class="left">
<li class="active"><a href="index.php?site=firstPage">Main</a></li>
<li class=""><a href="index.php?site=secondPage">Second</a></li>
</ul>
</section>
</nav>
</div>
<?php
include_once ("helper.php");
getContentSite("index");
?>
</body>
</html>
firstPage.php
<?php
$servername = "localhost";
$username = "root";
try {
$conn = new PDO("mysql:host=$servername;dbname=bs", $username);
// set the PDO error mode to exception
$conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
$sth = $conn->prepare("SELECT cus_vorname, cus_nachname FROM Customer;");
$sth->execute();
} catch (PDOException $e) {
echo "Connection failed: " . $e->getMessage();
}
?>
<!-- Filter -->
<input type="text" id="myInput" onkeyup="myFunction()" placeholder="Filter by Nachname">
<script>
function myFunction() {
var input, filter, table, tr, td, i, txtValue;
input = document.getElementById("myInput");
filter = input.value.toUpperCase();
table = document.getElementById("myTable");
tr = table.getElementsByTagName("tr");
for (i = 0; i < tr.length; i++) {
td = tr[i].getElementsByTagName("td")[1];
if (td) {
txtValue = td.textContent || td.innerText;
if (txtValue.toUpperCase().indexOf(filter) > -1) {
tr[i].style.display = "";
} else {
tr[i].style.display = "none";
}
}
}
}
</script>
<!-- Table generation -->
<table class="table" id="myTable">
<th>Vorname</th>
<th>Nachname</th>
<?php
while ($row = $sth->fetch(PDO::FETCH_ASSOC)) {
?>
<tr>
<td><?php echo $row['cus_vorname']; ?></td>
<td><?php echo $row['cus_nachname']; ?></td>
</tr>
<br>
<?php } ?>
</table>
<!-- Add New Customer To Table -->
<label> Add New Customer to Database</label><br>
<form action="index.php" method="POST">
<label>Vorname: </label><input type="text" name="vorname"><br>
<Label>Nachname:</Label><input type="text" name="nachname"><br>
<input type="submit">
</form>
<?php
if (isset($_POST['vorname']) && isset($_POST['nachname'])) {
$vorname = $_POST['vorname'];
$nachname = $_POST['nachname'];
$stmt = $conn->prepare('INSERT INTO Customer (cus_vorname, cus_nachname)
VALUES (?, ?)');
$stmt->execute([$vorname, $nachname]);
}
?>
<!-- Delete from Table -->
<label> Delete from Database via Nachname</label><br>
<form action="index.php" method="POST">
<Label>Nachname:</Label><input type="text" name="nachnameDelete"><br>
<input type="submit">
</form>
<?php
if (isset($_POST['nachnameDelete'])) {
$nachname = $_POST['nachnameDelete'];
$stmt = $conn->prepare('DELETE FROM Customer WHERE cus_nachname=?');
$stmt->execute([$nachname]);
}
?>
secondPage.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>Second Page</h1>
</body>
</html>